По экрану скачут шарики-изображения в случайном порядке
# This is the code for the balls example. It's a bit complicated, but most of
# this is the code for ball movement and so on. Only a very little bit of this
# actually deals with Ren'Py.
init python:
Import math
class Ball(object):
def __init__(self, filename, x, y, function=None):
self.transform = Transform(child=filename, xanchor=0.5, yanchor=0.5, rotate=0, function=function)
self.x = x
self.y = y
MAX_SPEED = 150
self.dx = renpy.random.uniform(-MAX_SPEED, MAX_SPEED)
self.dy = renpy.random.uniform(-MAX_SPEED, MAX_SPEED)
Rotation speed.
self.drotate = renpy.random.uniform(0, 180)
This is called
def balls_collide(p1, p2):
"""
Check to see if any of the balls are colliding. If they are,
Then handle the collision.
"""
DOUBLE_RADIUS = 75
x21 = p2.x - p1.x
y21 = p2.y - p1.y
d = math.hypot(x21, y21)
Return if too far.
if d > DOUBLE_RADIUS:
Return
vx21 = p2.dx - p1.dx
vy21 = p2.dy - p1.dy
Return if not approaching.
if (vx21 * x21 + vy21 * y21) > 0:
Return
Fix divide by zero.
if x21 == 0:
x21 = .00001
Compute the collision.
a = y21 / x21
dvx2 = -(vx21 + a * vy21) / (1 + a * a)
p2.dx += dvx2
p2.dy += a * dvx2
p1.dx -= dvx2
p2.dy -= a * dvx2
This is called by the first transform. It updates all of the
Transforms.
def balls_update(pilot, st, at):
Global last_time
RADIUS = 75 / 2
LEFT = RADIUS
RIGHT = 800 - RADIUS
TOP = RADIUS
BOTTOM = 600 - RADIUS
The pilot is the first ball in our list, and he's the one
That gets last_time updated.
if last_time is None:
dt = 0
else:
dt = st - last_time
last_time = st
Handle current collisions.
for i in xrange(0, len(balls)):
for j in xrange(i + 1, len(balls)):
balls_collide(balls[i], balls[j])
Basic movement, and bouncing off the walls.
for i in balls:
i.x += i.dx * dt
i.y += i.dy * dt
if i.x < LEFT:
i.x = LEFT
i.dx = abs(i.dx)
|
|
if i.x > RIGHT:
i.x = RIGHT
i.dx = -abs(i.dx)
if i.y < TOP:
i.y = TOP
i.dy = abs(i.dy)
if i.y > BOTTOM:
i.y = BOTTOM
i.dy = -abs(i.dy)
Update the transforms.
for i in balls:
This is the code that deals with Ren'Py to update the
Various transforms. Note that we use absolute coordinates
To position ourselves with subpixel accuracy.
i.transform.xpos = absolute(i.x)
i.transform.ypos = absolute(i.y)
i.transform.rotate = (i.drotate * st) % 360.0
I.transform.update()
Return 0
label start:
e "If you use it to do a rotation, you can zoom or adjust alpha at no additional cost."
hide logo base
with dissolve
python:
last_time = None
# Define a list of ball objects.
balls = [
Ball("eileen_orb.png", 200, 150, function=balls_update),
Ball("lucy_orb.png", 400, 150),
Ball("eileen_orb.png", 600, 150),
Ball("lucy_orb.png", 200, 300),
Ball("lucy_orb.png", 600, 300),
Ball("eileen_orb.png", 200, 450),
Ball("lucy_orb.png", 400, 450),
Ball("eileen_orb.png", 600, 450),
]
# Add each ball's transform to the screen.
for i, b in enumerate(balls):
renpy.show("ball%d" % i, what=b.transform)
with dissolve
e "As the python functions get more complicated, more advanced behavior is possible."
e "This can include coordinating more than one Transform."
python:
for i, b in enumerate(balls):
renpy.hide("ball%d" % i)
Движение и анимации
Самая простая анимация, моргание глаз. Для этого требуются 2 картинки. В 1 глаза закрыты, а в другой - они открыты.
|
|
Анимация моргания глаз.
· image ger_1:
· "images/1/1.png"
· pause 1.0
· "images/1/2.png"
· pause 1.0
· r epeat 2
· label start:
· show ger_1
или
· label start:
· scene ger_1
Теперь разберем что здесь записано
ger_1 – ссылка-метка
" images /1/1. png " – путь к 1 изображению
pause 1.0 – пауза длиной 1 секунда
" images /1/2. png " – 2 изображение, которое заменит 1
r epeat 2 – число повторений, если просто записать r epeat без цифр, то она будет бесконечно повторяться(Что не рекомендую делать, т.к. я прописал анимацию главного меню, каждую кнопку и т.д. запустил проект и пошел по «неотложным делам» минут на 5. Возвращаясь я увидел что новосозданный проект вывел на экран ошибку, мол ему, не хватило памяти, может быть со временем эту ошибку и исправили, но я все равно по старой привычке ставлю ограничение 30 повторений, как говорится на всякий случай
Дата добавления: 2018-10-27; просмотров: 693; Мы поможем в написании вашей работы! |
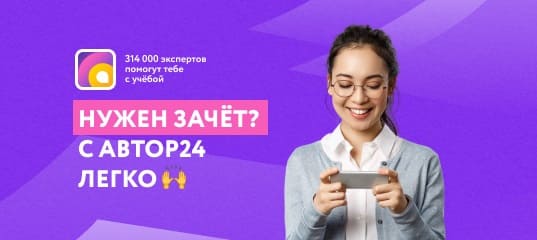
Мы поможем в написании ваших работ!