Напишите программу модуля RS триггера на языке Verilog
Приведите пример программного кода логических элементов на языке Verilog с помощью примитивов.
module (input in1, in2,output out1, out2, out3,out4);
and(out1, in1,in2); // логическое «И»
or(out2,in1,in2); //логическое «ИЛИ»
not(out3,in2); //логическое «НЕ»
xor(out4,in1,in2); // исключающееИЛИ
endmodule
primitive and _or(out, a1,a2,a3, b1,b2);
output out;
input a1,a2,a3, b1,b2;
table
//state table information goes here
0 | Логический 0 |
1 | Логическая 1 |
х | Не определено |
? | Любое значение из 0, 1 и x |
B | Итерация 0 и 1 |
0 0 0 0 0:0
0 1 0 0 0:1
endtable
endprimitive
Напишите программу на языке Verilog по ниже приведенной схеме
module shema (a, b, c, y);
input a, b, c;
output y;
wire d, e, f;
assign d= ~ b;
assign e=a & d;
assign f= b & c;
assign y=e | f;
endmodule
3.Напишите программу на языке Verilog для следующего алгебраического выражения F=(a+b)*c’+c*b
module ZZZ(input a, input b, input c, output F);
wire x,y;
assign x=(a|b)&(~c);
assign y=c&b;
assign F=x|y;
endmodule
4.Напишите программу на языке Verilog для следующего алгебраического выражения F=(a*c)’+(c+b) ’
module ZZZ(input a, input b, input c, output F);
wire x,y;
assign x=a&c;
assign y=c|b;
assign F=(~x)|(~y);
endmodule
Напишите программу модуля дешифратора 3x8 на языкеVerilog
module deshif (enable, in, out);
input enable;
input [2:0] in;
output reg [7:0] out;
always @(enable or in)
begin
out=0;
if (enable) begin
case(in)
3'b000: out=8'b00000001;
3'b001: out=8'b00000010;
3'b010: out=8'b00000100;
3'b011: out=8'b00001000;
3'b100: out=8'b00010000;
3'b101: out=8'b00100000;
3'b110: out=8'b01000000;
3'b111: out=8'b10000000;
endcase
end
end
endmodule
Напишите программу модуля дешифратора 2x4 на языке Verilog
module deshif (enable, in, out);
input enable;
input [1:0] in;
output reg [3:0] out;
|
|
always @(enable or in)
begin
out=0;
if (enable) begin
case(in)
2'b00:out=4'b0001;
2'b01:out=4'b0010;
2’b10:out=4’b0100;
2'b11:out=4'b1000;
endcase
end
end
endmodule
Напишите программу модуля шифратора 8x3 на языке Verilog
module deshif (enable, in, out);
input enable;
input [7:0] in;
output reg [2:0] out;
always @(enable or in)
begin
out=0;
if (enable) begin
case (in)
8'b10000000: out=3'b000;
8'b01000000: out=3'b001;
8'b00100000: out=3'b010;
8'b00010000: out=3'b011;
8'b00001000: out=3'b100;
8'b00000100: out=3'b101;
8'b00000010: out=3'b110;
8'b00000001: out=3'b111;
endcase
end
end
endmodule
Напишите программу модуля шифратора 4x2 на языке Verilog
module deshif (enable, in, out);
input enable;
input [3:0] in;
output reg [1:0] out;
always @(enable or in)
begin
out=0;
if (enable) begin
case(in)
4`b0001: out=2`b00;
4`b0010: out=2`b01;
4`b0100: out=2`b10;
4`b1000: out=2`b11;
endcase
end
end
endmodule
Напишите программу модуля мультиплексора 4x1 наязыкеVerilog
module multipleksor (input clk, input [3:0] in, input [1:0] addr, output reg out); //названиемодуля
always @ (posedge clk)
begin //начало
case (addr) //случай, выбор и т.д.
2’b00: out=in[0];
2’b01: out=in[1];
2’b10: out=in[2];
2’b11: out=in[3];
default out=0; //в других случаях
endcase //конец случая
end //конец
endmodule //конец модуля
|
|
Напишите программу модуля мультиплексора 8x1 на языке Verilog
module multipleksor (input clk, input [7:0] in, input [2:0] addr, output reg out);
always @ (posedge clk)
begin //начало
case (addr) //случай, выбор и т.д.
3’b000: out=in[0];
3’b001: out=in[1];
3’b010: out=in[2];
3’b011: out=in[3];
3’b100: out=in[4];
3’b101: out=in[5];
3’b110: out=in[6];
3’b111: out=in[7];
default out=0; //в других случаях
endcase //конец случая
end //конец
endmodule //конец модуля
Напишите программу модуля демультиплексора 1x8 на языке Verilog
module DEM (clk, addr, in, out);
input clk;
input reg [2:0] addr;
input in;
output reg [7:0] out;
always@(posedge clk)
begin if (in==1)
case(addr)
3’b000: begin out=0; out[0]=1; end
3’b001: begin out=0; out[1]=1; end
3’b010: begin out=0; out[2]=1; end
3’b011: begin out=0; out[3]=1; end
3’b100: begin out=0; out[4]=1; end
3’b101: begin out=0; out[5]=1; end
3’b110: begin out=0; out[6]=1; end
3’b111: begin out=0; out[7]=1; end
еndcase
еlse out=0;
еnd
еndmodule
Напишите программу модуля демультиплексора 1x4 наязыке Verilog
module DEM (clk, addr, in, out);
input clk;
input reg [1:0] addr;
input in;
output reg [3:0] out;
always@(posedge clk)
begin if (in==1)
case(addr)
2’b00: begin out=0; out[0]=1; end
2’b01: begin out=0; out[1]=1; end
|
|
2’b10: begin out=0; out[2]=1; end
2’b11: begin out=0; out[3]=1; end
еndcase
еlse out=0;
еnd
еndmodule
Напишите программу модуля D триггера на языке Verilog
module Dtrig ( a, b, clock, switch, reset, out );
input clock, reset ; // тактовая частота и сброс
input a, b; // входы
input switch; // управляющий сигнал
output out;
reg out;
wire in;
assign in = switch ? a : b;
always @ (posedge clock or posedge reset)
if (reset) out <=0;
else out<=in;
endmodule
Напишите программу модуля RS триггера на языке Verilog
module RS ( rs, clk, Q);
input [1:0] rs;
input clk;
output reg Q;
initial
begin
Q=1` b1;
end
always @ (posedge clk)
begin
if (rs==2` b00)
Q<=Q;
else
begin
if (rs==2` b01)
Q< =1` b1;
else
begin
if (rs==2` b10)
Q<=1` b0;
else
Q<=0;
end
end
end
endmodule
Дата добавления: 2018-06-01; просмотров: 1870; Мы поможем в написании вашей работы! |
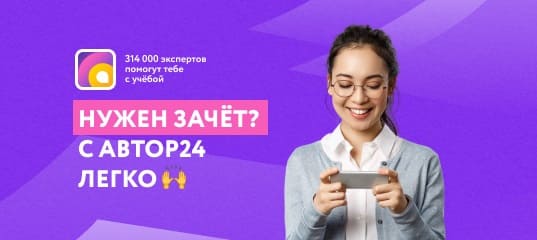
Мы поможем в написании ваших работ!