Перегрузка операций преобразования типов
Операции
Вопрос 1
Есть следующий код:
1 2 3 | int n1 = 2; int n2 = 5; int result = n2 * 3 + 20 / 2 * n1--; |
Используя приоритеты операций, разложите выражение int result = n2 * 3 + 20 / 2 * n1-- по шагам.
Вопрос 2
Есть следующий код:
1 2 3 4 5 6 7 8 | int num1 = 4; int num2 = 5; int num3 = 15; int num4 = 10; int num5 = 5; int result = 12; result += num1 * num2 + num3 % num4 / num5; |
Используя приоритеты операций, разложите выражение result += num1 * num2 + num3 % num4 / num5 по шагам.
Вопрос 3
Чему будет равна переменная z после выполнения следующего кода и почему?
1 2 3 | int x = 8; int y = 9; int z = x++ + ++y; |
Вопрос 4
Каккие арифметические операторы являются правоассоциативными?
Преобразование базовых типов
Вопрос 1
Какие преобразования типов НЕ выполняются автоматически (возможно, несколько вариантов):
Из int в short
Из short в int
Из bool в string
Из byte в float
Вопрос 2
Для чего нужен оператор checked?
Циклы
Вопрос 1
Сколько раз выполнится следующий цикл и почему:
1 2 3 4 5 6 | int i = 5; while(i > 0) { i *= 3; i *= -1; } |
Вопрос 2
Дан следующий цикл:
1 2 3 4 5 6 7 8 9 | int j = 2; for (int i = 1; i < 100; i = i + 2) { j = j - 1; while(j < 15) { j = j + 5; } } |
Сколько раз в этом цикле будет выполняться строка j = j - 1;
Вопрос 3
Дан следующий цикл:
1 2 3 4 5 6 7 8 9 | int j = 2; for (int i = 2; i < 32; i = i * 2) { while(i < j) { j = j * 2; } i = j - i; } |
Сколько раз в этом цикле будет выполняться строка i = j - i;
Вопрос 4
Что будет выведено на консоль в результате выполнения следующего цикла:
|
|
1 2 3 4 5 6 7 8 9 | for(int i = 1; i < 3; i++) { switch (i) { default: Console.WriteLine($"i = {i++}"); break; } } |
Варианты ответов:
a) Программа не скомпилируется
b) Ничего не будет выведено на консоль
c) Консоль будет иметь вывод
i = 1
d) Консоль будет иметь вывод
i = 1
i = 2
Массивы
Вопрос 1
Сколько элементов имеет следующий массив?
1 | int[,,] numbers = new int[3, 2, 3]; |
Варианты ответов
a) 0
b) 1
c) 8
d) 9
e) 11
f) 18
Вопрос 2
Сколько измерений (размерность) имеет следующий массив?
1 | int[,] numbers = new int[3, 3]; |
Варианты ответов
a) 1
b) 2
c) 3
d) 6
Вопрос 3
Что будет выведено на консоль в результате выполнения следующего кода:
1 2 3 4 5 6 | int[][] nums = new int[3][]; nums[0] = new int[2] { 1, 2 }; nums[1] = new int[3] { 3, 4, 5 }; nums[2] = new int[5] { 6, 7, 8, 9, 10 }; Console.WriteLine(nums[3][2]); |
Вопрос 4
Дан следующий массив
1 2 3 4 | int[][] nums = new int[3][]; nums[0] = new int[2] { 1, 2 }; nums[1] = new int[3] { 3, 4, 5 }; nums[2] = new int[5] { 6, 7, 8, 9, 10 }; |
Каким образом мы можем обратиться к числу 7 в этом массиве?
Варианты ответов
a) nums[7]
b) nums[2, 1]
c) nums[2][1]
d) nums[3]
e) nums[3, 3]
f) nums[3][3]
Параметры
Вопрос 1
Какой результат работы будет у следующей программы и почему:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | class Program { static void Main(string[] args) { int x; Increment(ref x); Console.WriteLine(x); Console.ReadKey(); } static void Increment(ref int x) { x++; } } |
Вопрос 2
|
|
Какое число (или какие числа) выведет на консоль следующая программа и почему:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | class Program { static void Main(string[] args) { int i = 0; Console.Write(i++ + Calculate(i)); Console.WriteLine(i); Console.ReadKey(); } public static int Calculate(int i) { Console.Write(i++); return i; } } |
Область видимости переменных
Вопрос 1
Какой результат работы будет у следующей программы и почему:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | class Program { static void Main(string[] args) { string message = "Hello"; Display(); Console.ReadKey(); } static void Display() { Console.WriteLine(message); } } |
Вопрос 2
Что будет выведено на консоль при выполнении следующей программы:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | class Program { int x = 8; static void Main(string[] args) { int x = 9; { x++; } Console.WriteLine(x); Console.ReadKey(); } } |
Варианты ответов:
a) 9
b) 10
c) 1
d) Программа не скомпилируется
Вопрос 3
Что будет выведено на консоль при выполнении следующей программы:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | class Program { int val = 8; static void Main(string[] args) { int val = 10; Doubled(ref val); Console.WriteLine(val); Console.ReadKey(); } static void Doubled(ref int val) { val *= 2; } } |
Варианты ответов:
|
|
a) 16
b) 20
c) 10
d) Программа не скомпилируется
Классы и объекты
Вопрос 1
Дан следующий класс:
1 2 3 4 5 6 7 8 9 10 11 | class Person { public string name = "Sam"; public int age; public Person(string name, int age) { this.name = name; this.age = age; } } |
Какое значение поле name будет иметь при выполнение следующего кода и почему?
1 | Person tom = new Person("Tom", 34) { name = "Bob", age = 29 }; |
Вопрос 2
Дан следующий класс:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | class Person { public string name = "Ben"; public int age = 18; public string email = "ben@gmail.com"; public Person(string name) { this.name = name; } public Person(string name, int age) : this(name) { this.age = age; } public Person(string name, int age, string email) : this("Bob", age) { this.email = email; } } |
Какое значения будут иметь поля name, age и email после выполнения следующего кода и почему? В каком порядке будут вызываться конструкторы класса Person?
1 | Person person = new Person("Tom", 31, "tom@gmail.com"); |
Структуры
Вопрос 1
Что будет выведено на консоль при выполнении следующей программы и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 | struct Person { public string name = "Sam"; } class Program { static void Main(string[] args) { Person person; person.name = "Bob"; Console.WriteLine(person.name); } } |
Вопрос 2
Почему не компилируется следующая программа:
|
|
1 2 3 4 5 6 7 8 9 10 11 12 13 | struct Person { public string name; } class Program { static void Main(string[] args) { Person person; Console.WriteLine(person.name); person.name = "Bob"; } } |
Вопрос 3
Почему не компилируется следующая программа:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | struct Person { public string name; public int age; public Person(string n) { name = n; } } class Program { static void Main(string[] args) { Person person; person.name = "Bob"; } } |
Модификаторы доступа
Вопрос 1
Какие модификаторы доступа есть в C# 7?
Вопрос 2
Вам надо определить в классе переменную, которая должна быть доступна из любого места в текущем проекте. Какой модификатор (или модификаторы, если их несколько) вы будете использовать?
Вопрос 3
В чем различие между модификаторами protected и private protected?
Вопрос 4
Если классы и члены класса не имеют никаких модификаторов, какие модификаторы доступа к ним применяются по умолчанию?
Вопрос 5
Что выведет на консоль следующая программа и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class Person { int age = 26; string name = "Tom"; public Person(int age, string name) { this.age = age; this.name = name; } } class Program { static void Main(string[] args) { Person person = new Person(19, "Bob"); Console.WriteLine(person.name); Console.ReadKey(); } } |
Свойства
Вопрос 1
Что будет выведено на консоль в результате выполнения следующей программы?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class Person { private int age = 15; public int Age { get { return age; } set { } } } class Program { static void Main(string[] args) { Person tom = new Person(); tom.Age = 25; Console.WriteLine(tom.Age); Console.ReadKey(); } } |
Варианты ответов:
a) 15
b) 25
c) 0
d) Программа не скомпилируется
Вопрос 2
Зачем нужны автосвойства, не проще ли их заменить обычными переменными?
Вопрос 3
Что будет выведено на консоль в результате выполнения следующей программы и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | class Person { internal string Name { get; set; } = "Bob"; } class Program { static void Main(string[] args) { Person tom = new Person { Name = "Tom" }; Console.WriteLine(tom.Name); Console.ReadKey(); } } |
Вопрос 4
Что будет выведено на консоль в результате выполнения следующей программы и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | class Person { internal string Name { internal get; set; } = "Bob"; } class Program { static void Main(string[] args) { Person tom = new Person { Name = "Tom" }; Console.WriteLine(tom.Name); Console.ReadKey(); } } |
Перегрузка методов
Вопрос 1
Каким образом можно перегрузить метод?
Вопрос 2
Корректна ли следующая перегрузка методов? Если да, то почему? Если нет, то почему?
1 2 3 4 5 6 7 8 9 10 11 | static void IncrementVal(ref int val) { val++; Console.WriteLine(val); } static void IncrementVal(int val) { val++; Console.WriteLine(val); } |
Статические члены класса
Вопрос 1
Какой результат будет у следующей программы и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | class Person { int retirementAge = 60; public static void ChangeRetirementAge(int years) { retirementAge += years; } public void DisplayRetirementAge() { Console.WriteLine(retirementAge); } } class Program { static void Main(string[] args) { Person tom = new Person(); Person.ChangeRetirementAge(5); tom.DisplayRetirementAge(); Console.Read(); } } |
Вопрос 2
Дана следующая программа:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | class Person { public static int retirementAge = 60; int _age; static Person() { Console.WriteLine($"Начальный пенсионный возраст: {retirementAge}"); } public Person(int age) { _age = age; } public void Display() { if (_age >= retirementAge) Console.WriteLine("Вы уже на пенсии"); else Console.WriteLine($"До пенсии осталось {retirementAge - _age} лет"); } } class Program { static void Main(string[] args) { Person tom; tom = new Person(34); Person.retirementAge = 65; tom.Display(); Console.ReadKey(); } } |
При выполнении какой строки кода в методе Main будет вызван конструктор класса Person?
Перегрузка операторов
Вопрос 1
Оператор + (сложение) при применении к двум объектам типа int возвращает их сумму.
1 2 3 | int x = 9; int y = 10; int z = x + y; // 19 |
Как можно переопределить оператор + для типа int, чтобы этот оператор возвращал не сумму, а произведение двух объектов int?
Вопрос 2
Есть класс Counter:
1 2 3 4 5 6 | class Counter { public int Number { get; set; } // определение оператора сложения } |
В этом классе определен оператор сложения, который позволяет складывать объект Counter с объектом типа int следующим образом:
1 2 3 | Counter counter = new Counter { Number = 45 }; int x = counter + 6; Console.WriteLine(x); // 51 |
Какой именно оператор сложения определен в классе Counter? Варианты ответов (правильных вариантов может быть несколько)
a)
1 2 3 4 | public int operator + (Counter counter, int val) { return counter.Number + val; } |
b)
1 2 3 4 | public static int operator + (Counter counter, int val) { return counter.Number + val; } |
c)
1 2 3 4 | public static int operator + (int val, Counter counter) { return counter.Number + val; } |
d)
1 2 3 4 | public static Counter operator + (Counter counter, int val) { return new Counter {Number = counter.Number + val}; } |
e)
1 2 3 4 | public static Counter operator + (int val, Counter counter) { return new Counter {Number = counter.Number + val}; } |
Вопрос 3
Почему не компилируется следующая программа?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | class Counter { public int Number { get; set; } public static int operator + (int val, Counter counter) { return counter.Number + val; } } class Program { static void Main(string[] args) { Counter counter = new Counter { Number = 45 }; int x = counter + 6; Console.WriteLine(x); // 51 Console.ReadKey(); } } |
Наследование
Вопрос 1
Почему следующая программа не компилируется:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | using System; namespace HelloApp { class Program { static void Main(string[] args) { Person tom = new Employee(); Console.ReadKey(); } } internal class Person { } public class Employee : Person { } } |
Вопрос 2
Даны следующие классы:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | class Person { string name; int age; public Person() { } public Person(string name) : this(name, 18) { } public Person(string name, int age) { this.name = name; this.age = age; } } class Employee : Person { string company; public Employee() { } public Employee(string name, int age, string company): base(name, age) { this.company = company; } public Employee(string name, string company) : base(name) { this.company = company; } } |
Допустим, мы создаем объект класса Employee следующим образом:
1 | Employee tom = new Employee("Tom", "Microsoft"); |
Какие конструкторы и в каком порядке в данном случае будет выполняться?
Вопрос 3
Как запретить наследование от класса?
Вопрос 4
Что выведет на консоль следующая программа и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | class Auto // легковой автомобиль { public int Seats { get; set; } // количество сидений public Auto(int seats) { Seats = seats; } } class Truck : Auto // грузовой автомобиль { public decimal Capacity { get; set; } // грузоподъемность public Truck(int seats, decimal capacity) { Seats = seats; Capacity = capacity; } } class Program { static void Main(string[] args) { Truck truck = new Truck(2, 1.1m); Console.WriteLine($"Грузовик с грузоподъемностью {truck.Capacity} тонн"); Console.ReadKey(); } } |
Вопрос 5
Что выведет на консоль следующая программа и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | class Auto // легковой автомобиль { public int Seats { get; set; } // количество сидений public Auto() { Console.WriteLine("Auto has been created"); } public Auto(int seats) { Seats = seats; } } class Truck : Auto // грузовой автомобиль { public decimal Capacity { get; set; } // грузоподъемность public Truck(decimal capacity) { Seats = 2; Capacity = capacity; Console.WriteLine("Truck has been created"); } } class Program { static void Main(string[] args) { Truck truck = new Truck(1.1m); Console.WriteLine($"Truck with capacity {truck.Capacity}"); Console.ReadKey(); } } |
Вопрос 6
Что выведет на консоль следующая программа и почему?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | class Person { public string Name { get; set; } = "Ben"; public Person(string name) { Name = "Tim"; } } class Employee : Person { public string Company { get; set; } public Employee(string name, string company) : base("Bob") { Company = company; } } class Program { static void Main(string[] args) { Employee emp = new Employee("Tom", "Microsoft") { Name = "Sam" }; Console.WriteLine(emp.Name); // Ben Tim Bob Tom Sam Console.ReadKey(); } } |
Преобразование типов
Вопрос 1
Что будет выведено на консоль в результате выполнения следующей программы и почему?:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | using System; namespace HelloApp { class Program { static void Main(string[] args) { Person tom = new Person { Name = "Tom" }; Employee empl = tom as Employee; tom.Name = "Bob"; Console.WriteLine(empl.Name); Console.ReadKey(); } } class Person { public string Name { get; set; } } class Employee : Person { } } |
Вопрос 2
Что будет выведено на консоль в результате выполнения следующей программы и почему?:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | using System; namespace HelloApp { class Program { static void Main(string[] args) { Person tom = new Employee { Name = "Tom" }; Person bob = tom; Employee empl = bob as Employee; tom.Name = "Bob"; Console.WriteLine(empl.Name); Console.ReadKey(); } } class Person { public string Name { get; set; } } class Employee : Person { } } |
Вопрос 3
Есть два класса:
1 2 3 4 5 | class Person { public string Name { get; set; } } class Employee : Person { } |
Для преобразования объекта Person к типу Employee мы можем использовать следующие два способа:
1 2 3 | Person tom = new Person { Name = "Tom" }; Employee empl1 = tom as Employee; Employee empl2 = (Employee)tom; |
Какой из способов является более предпочтительным и почему?
Перегрузка операций преобразования типов
Вопрос 1
Что выведет на консоль следующий код и почему:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class Clock { public int Hours { get; set; } public static explicit operator int(Clock clock) { return clock.Hours * 2; } } class Program { static void Main(string[] args) { Clock clock = new Clock { Hours = 15 }; int x = clock; Console.WriteLine(x); // 30 Console.ReadKey(); } } |
Вопрос 2
При проектировании следующих классов допущены ошибки. Какие?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | class Dollar { public decimal Sum { get; set; } } class Rouble { public decimal Sum { get; set; } public implicit operator Dollar(Rouble roubles) { return new Dollar { Sum = roubles.Sum / 66 }; } public implicit operator Rouble(Dollar dollars) { return new Rouble { Sum = dollars.Sum * 66 }; } public implicit operator decimal(Rouble roubles) { return roubles.Sum; } public implicit operator decimal(Dollar dollars) { return dollars.Sum; } } |
Варианты ответов (правильных вариантов может быть несколько):
a) Нельзя определить оператор преобразования в тип decimal
b) Нельзя определить все операторы преобразования как неявные (с ключевым словом implicit). Необходимо сделать один или несколько операторов явными (определить с ключевым словом explicit)
c) Слово operator лишнее, так как ключевые слова implicit и explicit уже говорят о том, что определяется оператор преобразования типов
d) Все операторы преобразования типов должны быть определены как статические (с ключевым словом static)
e) Необходимо добавить операторы преобразования типов также и в класс Dollar, иначе преобразования раотать не будут
f) Оператор преобразования типов должен преобразовывать из типа или в тип, в котором этот оператор определен.
Вопрос 3
Когда необходимо объявлять оператор преобразования как явный (с ключевым словом explicit), и когда как неявный (с ключевым словом implicit)?
Дата добавления: 2021-05-18; просмотров: 426; Мы поможем в написании вашей работы! |
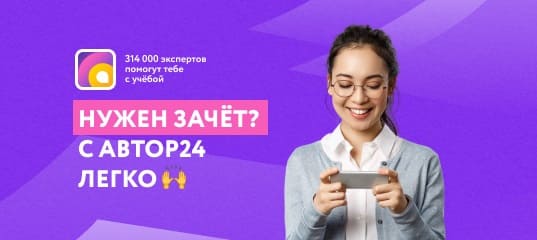
Мы поможем в написании ваших работ!