Получение характеристик строк
в классе string определено несколько функций-членов, позволяющих получить длину строки и объем памяти, занимаемый объектом:
size_type size () const; // Количество элементов строки
size_type length() const; // Количество элементов строки
size_type max_size() const; // Максимальная длина строки
size_type capacity() const; // Объем памяти, занимаемый строкой
bool empty() const; // Истина, если строка пустая
Программа
#include <stdio.h>
#include <ctype.h>
void DeletePhrases(FILE* f, char nameChanged[]);
void WriteToFile(FILE* fTo, FILE* fFrom, long posStart, long posFinal);
int main()
{
FILE* f;
char nameFile[ ] = "File 1.txt",
nameChanged[ ] = "File 1 (buf).txt";
f = fopen(nameFile, "r");
if (f == NULL)
{
printf("Unable to open file!\n");
return 1;
}
DeletePhrases(f, nameChanged);
fclose(f);
return 0;
}
// Удаление фраз, содержащих заглавную букву
void DeletePhrases(FILE* f, char nameChanged[])
{
FILE* fBuf;
long posStart = 0, // Позиция начала фразы
posFinal; // Позиция конца фразы
int c; // Считанный символ
char needDel = 0, // Нужно удалять фразу
wasRead = 0, // Файл не пуст
foundFirst = 0; // Найден первый символ фразы
fBuf = fopen(nameChanged, "w");
if (fBuf == NULL)
{
printf("Unable to open file!\n");
return;
}
while ((c = getc(f)) != EOF)
{
wasRead = 1;
posFinal = ftell(f) - 1;
if (!needDel)
{
if (isalpha(c)) // Символы внутри фразы
{
if (foundFirst)
{
if (c >= 'A' && c <= 'Z')
|
|
needDel = 1;
}
else
{
foundFirst = 1;
}
}
}
if (c == '.') // Конец фразы
{
if (!needDel)
WriteToFile(fBuf, f, posStart, posFinal);
posStart = posFinal + 1;
needDel = 0;
foundFirst = 0;
}
}
if (wasRead && !needDel)
WriteToFile(fBuf, f, posStart, posFinal);
fclose(fBuf);
}
// Запись фразы в файл
void WriteToFile(FILE* fTo, FILE* fFrom, long posStart, long posFinal)
{
int c;
fseek(fFrom, posStart, SEEK_SET);
while (ftell(fFrom) <= posFinal)
{
c = getc(fFrom);
putc(c, fTo);
}
}
2.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 10
int main()
{
FILE* fin, * fout_1, * fout_2, * fout_3;
int i, r, k;
char s[N], f[N];
int math, physics, philosophy;
fin = fopen("fin.txt", "rt");
fout_1 = fopen("fout_1.txt", "wt");
fout_2 = fopen("fout_2.txt", "wt");
fout_3 = fopen("fout_3.txt", "wt");
if (fin == NULL || fout_1 == NULL || fout_2 == NULL || fout_3 == NULL)
return 1;
k = 0;
while (!feof(fin)) // а теперь пока не конец файла
{
for (i = 0; i < 5; i++)
{
if (i == 0)
{
fscanf(fin, "%s", s);
strcpy(f, s);
}
if (i == 1)
{
fscanf(fin, "%s", s);
}
if (i != 0 && i != 1)
{
fscanf(fin, "%d", &r);
if (i == 2)
math = r;
if (i == 3)
physics = r;
if (i == 4)
philosophy = r;
}
k++;
|
|
}
if (math == 5 && physics == 5 && philosophy == 5)
fprintf(fout_1, "%s\n", f);
if ((math != 3 && physics != 3 && philosophy != 3)&&(math == 4 || physics == 4 || philosophy == 4))
fprintf(fout_2, "%s\n", f);
if (math == 3 || physics == 3 || philosophy == 3)
fprintf(fout_3, "%s\n", f);
}
k = k / 5;
printf("%d\n", k);
fclose(fin);
fclose(fout_1);
fclose(fout_2);
fclose(fout_3);
system("pause");
return 0;
}
3.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <math.h>
#include <conio.h>
#include <process.h>
#include <time.h>
#include <stdlib.h>
#include <locale.h>
#define N 10
void datfilecreate(void);
int main()
{
int p,k = 0, i, n = 0, X[N], x = 0;
setlocale(LC_ALL, "Russian");
FILE* fin, * fout;
srand(time(0));
datfilecreate();
fin = fopen("text.dat", "rb");
fout = fopen("text_new.txt", "w+t");
if (!fin || !fout)
{
printf("ERROR - file not open or write\n");
system("pause");
exit(1);
}
while (fread(&n, sizeof(int), 1, fin))
{
X[k] = n;
printf("%d\t", n);
k++;
x += n;
}
printf("\n\n сумма %d \n", x);
printf("количество %d\n", k);
x = x / k;
printf("среднее число %d\n", x);
p = (x/3);
printf("от %d и до %d\n", p ,3*x);
for (i = 0;i < N;i++)
{
if (X[i] > p && X[i] < 3 * x)
X[i] = 0;
}
for (i = 0;i < N;i++)
printf("%d\t", X[i]);
printf("\n");
for (i = 0;i < N;i++)
{
fprintf(fout, " %d;", X[i]);
printf("%d\t", X[i]);
}
printf("\n");
fclose(fin);
fclose(fout);
system("pause");
return 0;
}
void datfilecreate(void)
{
int i = 0, n;
FILE* f = fopen("text.dat", "wb");
if (!f)
{
printf("ERROR - file not create\n");
system("pause");
exit(1);
}
while (i < N
n = rand() % 101 ;
fwrite(&n, sizeof(int);
i++;
}
fclose(f);
}
|
|
Список литературы
1. Лебедев И.С., Петров В.Ю. Информатика. Програмирование: часть 2/ ИТМО. – СПб: 2017. 73 c.
2. Основы программирования [электронный ресурс] – https://informatica-c.jimdo.com/семестр-ii/ ( дата обращения – 01.01.2017 г.).
3. Шилдт, Герберт полный справочник по C#. : Пер. с англ. — М. : Издательский дом “Вильямс”, 2004. — 752 с. : ил. — Парал. тит. англ.
4. C/C++. Программирование на языке высокого уровня / Т. А. Павловская. — СПб.: Питер, 2003. —461 с:
Дата добавления: 2021-07-19; просмотров: 72; Мы поможем в написании вашей работы! |
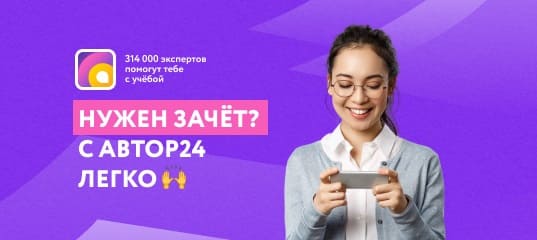
Мы поможем в написании ваших работ!