How to: Read Characters from a String
The following code example allows you to read a certain number of characters from an existing string, starting at a specified place in the string. Use StringReader to do this, as demonstrated below.
This code defines a string and converts it to an array of characters, which can then be read by using the appropriate StringReader..::.Read method.
This example reads only the specified number of characters from the string, as follows.
Some number o |
Example
using System; using System.IO; public class CharsFromStr { public static void Main(String[] args) { // Create a string to read characters from. String str = "Some number of characters"; // Size the array to hold all the characters of the string // so that they are all accessible. char[] b = new char[24]; // Create an instance of StringReader and attach it to the string. StringReader sr = new StringReader(str); // Read 13 characters from the array that holds the string, starting // from the first array member. sr.Read(b, 0, 13); // Display the output. Console.WriteLine(b); // Close the StringReader. sr.Close(); } } |
Считывание символов из строки
С помощью приведенного ниже примера кода можно считать некоторое количество символов из существующей строки, начиная с указанной позиции в строке. Для этого, как показано ниже, используется StringReader.
Этот код определяет строку и преобразует ее в массив символов, который потом может быть считан с использованием соответствующего метода StringReader..::.Read.
Этот пример считывает только указанное количество символов из строки.
Пример
ß----------
Результат
Some number o
How to: Write Characters to a String
The following code example writes a certain number of characters from a character array into an existing string, starting at a specified place in the array. Use StringWriter to do this, as demonstrated below.
Example
using System; using System.IO; using System.Text; public class CharsToStr { public static void Main(String[] args) { // Create an instance of StringBuilder that can then be modified. StringBuilder sb = new StringBuilder("Some number of characters"); // Define and create an instance of a character array from which // characters will be read into the StringBuilder. char[] b = {' ','t','o',' ','w','r','i','t','e',' ','t','o','.'}; // Create an instance of StringWriter // and attach it to the StringBuilder. StringWriter sw = new StringWriter(sb); // Write three characters from the array into the StringBuilder. sw.Write(b, 0, 3); // Display the output. Console.WriteLine(sb); // Close the StringWriter. sw.Close(); } } |
Robust Programming
|
|
This example illustrates the use of a StringBuilder to modify an existing string. Note that this requires an additional using declaration, since the StringBuilder class is a member of the System.Text namespace. Also, instead of defining a string and converting it to a character array, this is an example of creating a character array directly and initializing it.
This code produces the following output.
Some number of characters to |
Запись символов в строку
С помощью приведенного ниже примера кода можно записать определенное количество символов из массива символов в существующую строку, начиная с указанной позиции в этом массиве. Для этого, как показано ниже, используется StringWriter.
Пример
ß--------
Надежное программирование
Этот пример иллюстрирует использование StringBuilder для изменения существующей строки. Обратите внимание, что это требует дополнительной декларации using, поскольку класс StringBuilder является членом пространства имен System.Text. Вместо того чтобы определять строку и затем преобразовывать ее в массив символов, с помощью примера можно напрямую создать и инициализировать массив символов.
|
|
В результате получаются следующие выходные данные.
Some number of characters to |
How to: Add or Remove Access Control List Entries
To add or remove Access Control List (ACL) entries to or from a file, the FileSecurity or DirectorySecurity object must be obtained from the file or directory, modified, and then applied back to the file or directory.
Дата добавления: 2019-03-09; просмотров: 215; Мы поможем в написании вашей работы! |
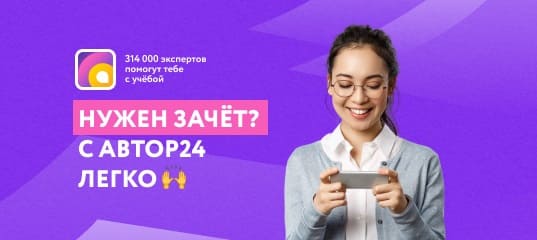
Мы поможем в написании ваших работ!