How to: Identify a Nullable Type
You can use the C# typeof operator to create a Type object that represents a Nullable type:
System.Type type = typeof(int?); |
You can also use the classes and methods of the System.Reflection namespace to generate Type objects that represent Nullable types. However, if you try to obtain type information from Nullable variables at runtime by using the GetType method or the is operator, the result is a Type object that represents the underlying type, not the Nullable type itself.
Calling GetType on a Nullable type causes a boxing operation to be performed when the type is implicitly converted to Object. Therefore GetType always returns a Type object that represents the underlying type, not the Nullable type.
int? i = 5; Type t = i.GetType(); Console.WriteLine(t.FullName); //"System.Int32" |
The C# is operator also operates on a Nullable's underlying type. Therefore you cannot use is to determine whether a variable is a Nullable type. The following example shows that the is operator treats a Nullable<int> variable as an int.
static void Main(string[] args) { int? i = 5; if (i is int) // true //… } |
Example
Use the following code to determine whether a Type object represents a Nullable type. Remember that this code always returns false if the Type object was returned from a call to GetType, as explained earlier in this topic.
if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>)) {…} |
Идентификация типа, допускающего значение null
Оператор C# typeof может использоваться для создания объекта Type, представляющего тип, допускающий значение null:
System.Type type = typeof(int?); |
Также можно использовать классы и методы пространства имен System.Reflection для создания объектов Type, представляющих типы, допускающие значение null. Однако при попытке получить информацию о типе от переменных типов, допускающих значение null , во время выполнения, используя метод GetType или оператор is, результатом будет объект Type, представляющий базовый тип, а не сам обнуляемый тип.
|
|
Вызов GetType для типа, допускающего значение null, приводит к выполнению операции упаковки-преобразования в момент неявного преобразования типа в Object. Таким образом, GetType всегда возвращает объект Type, представляющий базовый тип, а не обнуляемый тип.
ß----
Оператор C# is также работает с базовым типом для обнуляемого типа. Поэтому нельзя использовать is для определения, является ли тип переменной обнуляемым. В следующем примере показано, что оператор is рассматривает обнуляемую переменную<int> просто как "int".
ß---
Пример
Чтобы определить, представляет ли объект Type обнуляемый тип, используйте следующий код. Помните, что код всегда возвращает значение "false", если объект Type был возвращен из вызова GetType, как описано ранее в этом разделе.
if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>)) {…} |
Unsafe Code and Pointers
To maintain type safety and security, C# does not support pointer arithmetic, by default. However, by using the unsafe keyword, you can define an unsafe context in which pointers can be used.
![]() |
In the common language runtime (CLR), unsafe code is referred to as unverifiable code. Unsafe code in C# is not necessarily dangerous; it is just code whose safety cannot be verified by the CLR. The CLR will therefore only execute unsafe code if it is in a fully trusted assembly. If you use unsafe code, it is your responsibility to ensure that your code does not introduce security risks or pointer errors. |
Unsafe Code Overview
|
|
Unsafe code has the following properties:
· Methods, types, and code blocks can be defined as unsafe.
· In some cases, unsafe code may increase an application's performance by removing array bounds checks.
· Unsafe code is required when you call native functions that require pointers.
· Using unsafe code introduces security and stability risks.
· In order for C# to compile unsafe code, the application must be compiled with /unsafe.
Небезопасный код и указатели
Для обеспечения строгой типизации и безопасности C# по умолчанию не поддерживает арифметические операции над указателями. Однако с помощью ключевого слова unsafe можно определить небезопасный контекст для использования указателей. Дополнительные сведения об указателях см. в разделе Типы указателей.
Дата добавления: 2019-03-09; просмотров: 213; Мы поможем в написании вашей работы! |
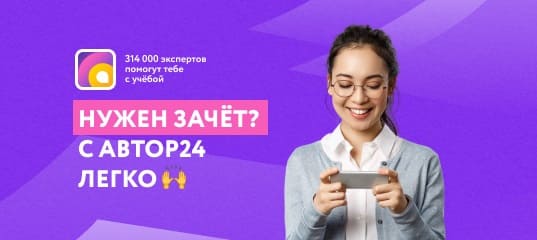
Мы поможем в написании ваших работ!