How to: Implement User-Defined Conversions Between Structs
This example defines two structs, RomanNumeral and BinaryNumeral, and demonstrates conversions between them.
Example
struct RomanNumeral { private int value; public RomanNumeral(int value) //constructor { this.value = value; } static public implicit operator RomanNumeral(int value) { return new RomanNumeral(value); } static public implicit operator RomanNumeral(BinaryNumeral binary) { return new RomanNumeral((int)binary); } static public explicit operator int(RomanNumeral roman) { return roman.value; } static public implicit operator string(RomanNumeral roman) { return ("Conversion not yet implemented"); } } |
Реализация определенных пользователем преобразований между структурами
В данном примере определяются две структуры RomanNumeral и BinaryNumeral и демонстрируется преобразование между ними.
Пример
ß---
struct BinaryNumeral { private int value; public BinaryNumeral(int value) //constructor { this.value = value; } static public implicit operator BinaryNumeral(int value) { return new BinaryNumeral(value); } static public explicit operator int(BinaryNumeral binary) { return (binary.value); } static public implicit operator string(BinaryNumeral binary) { return ("Conversion not yet implemented"); } } class TestConversions { static void Main() { RomanNumeral roman; BinaryNumeral binary; roman = 10; // Perform a conversion from a RomanNumeral to a BinaryNumeral: binary = (BinaryNumeral)(int)roman; // Perform a conversion from a BinaryNumeral to a RomanNumeral: // No cast is required: roman = binary; System.Console.WriteLine((int)binary); System.Console.WriteLine(binary); } } |
10 Conversion not yet implemented |
ß----
Robust Programming
· In the previous example, the statement:
binary = (BinaryNumeral)(int)roman; |
· performs a conversion from a RomanNumeral to a BinaryNumeral. Because there is no direct conversion from RomanNumeral to BinaryNumeral, a cast is used to convert from a RomanNumeral to an int, and another cast to convert from an int to a BinaryNumeral.
|
|
· Also the statement
roman = binary; |
· performs a conversion from a BinaryNumeral to a RomanNumeral. Because RomanNumeral defines an implicit conversion from BinaryNumeral, no cast is required.
Надежное программирование
· В предыдущем примере оператор
binary = (BinaryNumeral)(int)roman; |
· выполняет преобразование из RomanNumeral в BinaryNumeral. Поскольку прямого преобразования из RomanNumeral в BinaryNumeral не существует, используется приведение для преобразования из RomanNumeral в int и другое приведение для преобразования из int в BinaryNumeral.
· Кроме того, оператор
roman = binary; |
· выполняет преобразование из BinaryNumeral в RomanNumeral. Поскольку RomanNumeral определяет неявное преобразование из BinaryNumeral, приведение не требуется.
How to: Use Operator Overloading to Create a Complex Number Class
This example shows how you can use operator overloading to create a complex number class Complex that defines complex addition. The program displays the imaginary and the real parts of the numbers and the addition result using an override of the ToString method.
Example
Дата добавления: 2019-03-09; просмотров: 210; Мы поможем в написании вашей работы! |
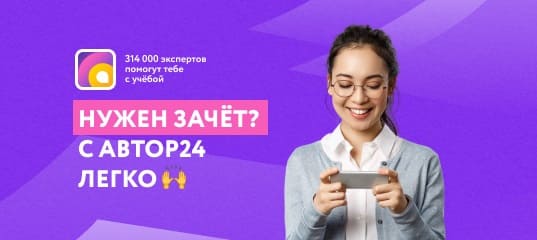
Мы поможем в написании ваших работ!