How to: Perform String Manipulations by Using Basic String Operations
The following example uses some of the methods discussed in the Basic String Operations topics to construct a class that performs string manipulations in a manner that might be found in a real-world application. The MailToData class stores the name and address of an individual in separate properties and provides a way to combine the City, State, and Zip fields into a single string for display to the user. Furthermore, the class allows the user to enter the city, state, and ZIP Code information as a single string; the application automatically parses the single string and enters the proper information into the corresponding property.
For simplicity, this example uses a console application with a command-line interface.
Example
using System; class MainClass { static void Main(string[] args) { MailToData MyData = new MailToData(); Console.Write("Enter Your Name:"); MyData.Name = Console.ReadLine(); Console.Write("Enter Your Address:"); MyData.Address = Console.ReadLine(); Console.Write( "Enter Your City, State, and ZIP Code separated by spaces:"); MyData.CityStateZip = Console.ReadLine(); Console.WriteLine("Name: {0}", MyData.Name); Console.WriteLine("Address: {0}", MyData.Address); Console.WriteLine("City: {0}", MyData.City); Console.WriteLine("State: {0}", MyData.State); Console.WriteLine("Zip: {0}", MyData.Zip); Console.WriteLine("The following address will be used:"); Console.WriteLine(MyData.Address); Console.WriteLine(MyData.CityStateZip); } } |
Выполнение действия со строками с помощью основных строковых операций
В следующем примере некоторые методы, описанные в разделе Основные операции со строками, используются для создания класса, который выполняет обработку строк так же, как это происходит в реальном приложении. Класс MailToData хранит имя и адрес человека в отдельных свойствах и предоставляет способ объединения полей City, State и Zip в одну строку для отображения пользователю. Более того, данный класс позволяет пользователю вводить сведения о городе, области и почтовом индексе в одну строку. Приложение автоматически разбирает эту строку и вводит необходимые сведения в соответствующее свойство.
|
|
В этом упрощенном примере используется консольное приложение с интерфейсом командной строки.
Пример
ß------------
public class MailToData
{
string name = " ";
string address = " ";
string citystatezip = " ";
string city = " ";
string state = " ";
string zip = " ";
public MailToData()
{
}
public string Name
{
get{return name;}
set{name = value;}
}
public string Address
{
get{return address;}
set{address = value;}
}
public string CityStateZip
{
get
{
return ReturnCityStateZip();
}
set
{
citystatezip = value;
ParseCityStateZip();
}
}
public string City
{
get{return city;}
set{city = value;}
}
public string State
{
get{return state;}
set{state = value;}
}
public string Zip
{
get{return zip;}
set{zip = value;}
}
ß-----------
private void ParseCityStateZip()
{
int CityIndex;
int StateIndex;
// Check for an exception if the user did not enter spaces between
// the elements.
try
{
// Find index position of the space between
// city and state and assign that value to CityIndex.
CityIndex = citystatezip.IndexOf(" ");
// Initialize the CityArray to the value of the
// index position of the first white space.
|
|
char[] CityArray = new char[CityIndex];
// Copy the city to the CityArray.
citystatezip.CopyTo(0,CityArray ,0, CityIndex);
// Find index position of the space between
// state and zip and assign that value to CityIndex.
StateIndex = citystatezip.LastIndexOf(" ");
// Initialize the StateArray to the length of the state.
char[] StateArray = new char[StateIndex - CityIndex];
// Copy the state to the StateArray.
citystatezip.CopyTo(CityIndex, StateArray, 0, (StateIndex - CityIndex));
// Initialize the ZipArray to the length of the zip.
char[] ZipArray = new char[citystatezip.Length - StateIndex];
// Copy the zip to the ZipArray.
citystatezip.CopyTo(StateIndex, ZipArray, 0, (citystatezip.Length - StateIndex));
// Assign city to the value of CityArray.
city = new String(CityArray);
// Trim white spaces, commas, and so on.
city = city.Trim(new char[]{' ', ',', ';', '-', ':'});
// Assign state to the value of StateArray.
state = new String(StateArray);
// Trim white spaces, commas, and so on.
state = state.Trim(new char[]{' ', ',', ';', '-', ':'});
// Assign zip to the value of ZipArray.
zip = new String(ZipArray);
// Trim white spaces, commas, and so on.
zip = zip.Trim(new char[]{' ', ',', ';', '-', ':'});
}
// If an exception is encountered, alert the user to enter spaces
// between the elements.
catch(OverflowException)
{
Console.WriteLine("\n\nYou must enter spaces between elements.\n\n");
}
}
ß---------------
private string ReturnCityStateZip() { // Make state uppercase. state = state.ToUpper(); // Put the value of city, state, and zip together //in the proper manner. string MyCityStateZip = String.Concat(city, ", ", state, " ", zip); return MyCityStateZip; } } |
When the preceding code is executed, the user is asked to enter his or her name and address. The application places the information in the appropriate properties and displays the information back to the user, creating a single string that displays the city, state, and ZIP Code information.
|
|
ß------
При выполнении предыдущего кода пользователю предлагается ввести его имя и адрес. Приложение размещает сведения в соответствующих свойствах и отображает их для пользователя, создавая одну строку, в которой представлены сведения о городе, области и почтовом индексе.
Parsing Strings
The Parse method converts a string that represents a .NET Framework base type into an actual .NET Framework base type. Because parsing is the reverse operation of formatting (converting a base type into a string representation), many of the same rules and conventions apply. Just as formatting uses an object that implements the IFormatProvider interface to format the information into a string, parsing also uses an object that implements the IFormatProvider interface to determine how to interpret a string representation.
Parsing Numeric Strings
All numeric types have a static Parse method that you can use to convert a string representation of a numeric type into an actual numeric type. These methods allow you to parse strings that were produced using one of the formatting specifiers covered in Numeric Format Strings.
The characters used to represent currency symbols, thousand separators, and decimal points are defined in format providers. The Parse method accepts a format provider, allowing you to specify and explicitly parse culture-specific strings. If no format provider is specified, then the provider associated with the current thread is used.
|
|
The following code example converts a string to an integer value, increments that value, and displays the result.
string MyString = "12345"; int MyInt = int.Parse(MyString); MyInt++; Console.WriteLine(MyInt); // The result is "12346". |
Разбор строк
Метод Parse преобразует строку, представляющую базовый тип .NET Framework, в реальный базовый тип .NET Framework. Поскольку разбор — это операция, обратная форматированию (преобразования базового типа в строковое представление), то применимы многие схожие правила и условия. Подобно тому, как при форматировании используется объект, реализующий интерфейс IFormatProvider для форматирования данных в строку, точно так же и при разборе используется объект, реализующий интерфейс IFormatProvider для преобразования в строковое представление.
Разбор числовых строк
Для всех числовых типов имеется статический метод Parse, который можно использовать для преобразования строкового представления числового типа в реальный числовой тип. Эти методы позволяют разбирать строки, которые были созданы с помощью одного из спецификаторов формата в разделе Строки числовых форматов.
Знаки, используемые для представления символов денежной единицы, разделителей групп разрядов и разделителей целой и дробной частей числа, описываются в поставщиках формата. Метод Parse допускает применение поставщика формата, что позволяет задавать и выполнять явный разбор строк, связанных с региональными параметрами. Если поставщик формата не указан, то используется поставщик, связанный с текущим потоком.
В следующем примере кода выполняется преобразование строки в целочисленное значение, увеличение этого значения и вывод результата на экран.
string MyString = "12345"; int MyInt = int.Parse(MyString); MyInt++; Console.WriteLine(MyInt); // The result is "12346". |
The NumberStyles enumeration indicates the permitted format of a string to parse. You can use this enumeration to parse a string that contains a currency symbol, a decimal point, an exponent, parentheses, and so on. For example, in the en-US culture, a string that contains a comma cannot be converted to an integer value using the Parse method if the NumberStyles.AllowThousands enumeration is not passed.
NumberStyles.AllowCurrencySymbol specifies that a number should be parsed as a currency rather than as a decimal. NumberStyles.AllowDecimalPoint indicates that a decimal point is allowed. Valid decimal point characters are determined by the NumberDecimalSeparator or CurrencyDecimalSeparator properties of the current NumberFormatInfo object. NumberStyles.AllowThousands indicates that group separators are allowed. Valid group separator characters are determined by the NumberGroupSeparator or CurrencyGroupSeparator properties of the current NumberFormatInfo object. For a complete table of valid nonnumeric character types, see the NumberStyles enumeration documentation.
The NumberStyles enumeration uses the characters specified by the current culture to aid in the parse. If you do not specify a culture by passing a CultureInfo object set to the culture that corresponds to the string you are parsing, the culture associated with the current thread is used.
The following code example is invalid and will raise an exception. It illustrates the improper way to parse a string containing nonnumeric characters. A new CultureInfo is first created and passed to the Parse method to specify that the en-US culture be used for parsing.
using System.Globalization; CultureInfo MyCultureInfo = new CultureInfo("en-US"); string MyString = "123,456"; int MyInt = int.Parse(MyString, MyCultureInfo); Console.WriteLine(MyInt); // Raises System.Format exception. |
Перечисление NumberStyles указывает разрешенный формат разбираемой строки. Это перечисление можно использовать для разбора строки, содержащей знак денежной единицы, разделитель целой и дробной частей числа, степень, скобки и т. д. Например, в региональных параметрах en-US строка, содержащая запятую, не может быть преобразована в целое число с использованием метода Parse, если не будет передано перечисление NumberStyles.AllowThousands.
NumberStyles.AllowCurrencySymbol указывает, что число должно разбираться как денежная единица, а не как десятичное число. NumberStyles.AllowDecimalPoint показывает, что разрешен десятичный разделитель. Допустимые знаки десятичного разделителя определены свойствами NumberDecimalSeparator или CurrencyDecimalSeparator текущего объекта NumberFormatInfo. NumberStyles.AllowThousands показывает, что разрешен разделитель групп разрядов. Допустимые знаки разделителей групп разрядов определены свойствами NumberGroupSeparator или CurrencyGroupSeparator текущего объекта NumberFormatInfo. Полную таблицу допустимых типов нечисловых знаков см. в документации на перечисление NumberStyles.
В перечислении NumberStyles используются знаки, описанные в текущих региональных параметрах. Это перечисление используется при разборе. Если региональные параметры не описаны путем передачи объекта CultureInfo, устанавливающего параметры, соответствующие разбираемой строке, то используются региональные параметры, связанные с текущим потоком.
Следующий пример кода является недопустимым и поэтому будет создавать исключение. Этот пример описывает неправильный способ разбора строки, содержащей нечисловые знаки. Сначала создается объект CultureInfo, передаваемый в метод Parse, чтобы указать, что для разбора будут использованы региональные параметры en-US.
ß-------------
When you apply the NumberStyles enumeration with the AllowThousands flag, the Parse method ignores the comma that raised the exception in the previous example. The following code example uses the same string as the previous example, but does not raise an exception. Similar to the previous example, a new CultureInfo is first created and passed to the Parse method to specify that the thousand separator used by the en-US culture is used for parsing.
using System.Globalization; CultureInfo MyCultureInfo = new CultureInfo("en-US"); string MyString = "123,456"; int MyInt = int.Parse(MyString, NumberStyles.AllowThousands, MyCultureInfo); Console.WriteLine(MyInt); // The result is "123456". |
При использовании перечисления NumberStyles с флагом AllowThousands метод Parse не обрабатывает запятую, которая приводила к созданию исключения в предыдущем примере. В следующем примере кода используется та же самая строка, что и в предыдущем, но исключение не создается. Как и в предыдущем примере, сначала создается новый объект CultureInfo, передаваемый в метод Parse для того, чтобы указать, что для разбора будет использован разделитель групп разрядов, применяющийся в региональных параметрах en-US.
using System.Globalization; CultureInfo MyCultureInfo = new CultureInfo("en-US"); string MyString = "123,456"; int MyInt = int.Parse(MyString, NumberStyles.AllowThousands, MyCultureInfo); Console.WriteLine(MyInt); // The result is "123456". |
Дата добавления: 2019-03-09; просмотров: 218; Мы поможем в написании вашей работы! |
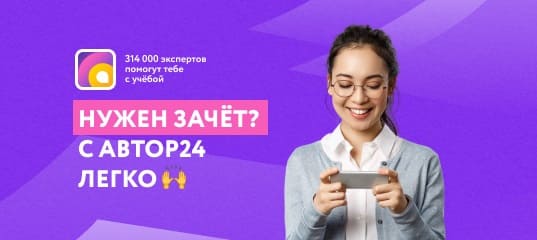
Мы поможем в написании ваших работ!