Сохранение записи через FileChooser
То же самое, что и в предыдущем примере, только для записи файла. Код неизвестных методов приводится ниже.
@FXML
private void saveItem(ActionEvent event){
if("".equals(t.getText())){
toast("Ничего нет для сохранения");
return;
}
FileChooser fileChooser = new FileChooser();
fileChooser.setTitle("Сохранение записи");
fileChooser.setInitialDirectory(new File(path));
fileChooser.setInitialFileName(dateToDay("Запись_от_dd_MMMM_yyyy_в_HH‑mm‑ss"));
File file = fileChooser.showSaveDialog(null);
if (file != null) {
recordInFile(t.getText(),file.getAbsolutePath(),false);
if("все заметки".equals(file.getName()))return;
recordInFile("\n"+file.getName()+" ("+dayOfWeek()+")"+"\n"+
t.getText(),path+System.getProperty("file.separator")+"все заметки", true);
label3.setText(file.getName());
}
}
Чтение и запись файла
void recordInFile(String text,String fileName,boolean b){
try(FileWriter sw = new FileWriter(fileName,b)) {
sw.write(text+"\n");
if(!b)
toast("Запись сохранена");
}catch(Exception e){
toast("Ошибка записи файла");
}
}
String readerFile(String s){
String str,f="";
try{
File file=new File(s);
FileReader fr=new FileReader(file);
BufferedReader br=new BufferedReader(fr);
while((str = br.readLine()) != null){
f+=str+"\n";
}
}catch(IOException e){
toast("Ошибка чтения файла");
}
return f;
}
Окно выхода из программы
@FXML
private void exitItem(ActionEvent event){
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.setTitle("ВЫХОД");
alert.setHeaderText("Выход из программы");
alert.setContentText("Вы действительно хотите выйти из программы?");
Optional<ButtonType> resultAlert = alert.showAndWait();
if (resultAlert.get() == ButtonType.OK){
System.exit(0);
}
}
Определение даты и дня недели
|
|
String dateToDay(String s){
Calendar calendar=new GregorianCalendar();
SimpleDateFormat sdf = new SimpleDateFormat(s);
return sdf.format(calendar.getTime());
}
String dayOfWeek(){
Calendar calendar = Calendar.getInstance();
String s="";
switch(calendar.get(Calendar.DAY_OF_WEEK)){
case 1:
s="воскресенье";
break;
case 2:
s="понедельник";
break;
case 3:
s="вторник";
break;
case 4:
s="среда";
break;
case 5:
s="четверг";
break;
case 6:
s="пятница";
break;
case 7:
s="суббота";
break;
default:
break;
}
return s;
}
Всплывающее сообщение как в Android(Toast)
Этот метод можно встретить в вышеприведенных примерах. Попробуйте его в своих проектах и получите практически полную копию всплывающего сообщения как в android.
void toast(String toastMsg){
Stage toastStage=new Stage();
toastStage.setResizable(false);
toastStage.initStyle(StageStyle.TRANSPARENT);
Text text = new Text(toastMsg);
text.setFont(Font.font("Verdana", 20));
text.setFill(Color.WHITE);
StackPane root = new StackPane(text);
root.setStyle("‑fx‑background‑radius: 10; ‑fx‑background‑color: rgba(0, 0, 0, 0.2); ‑fx‑padding: 20px;");
root.setOpacity(0);
Scene scene = new Scene(root);
scene.setFill(Color.BLACK);
toastStage.setScene(scene);
toastStage.show();
Timeline tl1 = new Timeline();
KeyFrame fadeInKey1 = new KeyFrame(Duration.millis(500), new KeyValue (toastStage.getScene().getRoot().opacityProperty(), 1));
tl1.getKeyFrames().add(fadeInKey1);
tl1.setOnFinished((ae) ‑>
{
new Thread(() ‑> {
try
{
Thread.sleep(2000);
}
catch (InterruptedException e)
{
e.getMessage();
}
Timeline tl2 = new Timeline();
|
|
KeyFrame fadeOutKey1 = new KeyFrame(Duration.millis(500), new KeyValue (toastStage.getScene().getRoot().opacityProperty(), 0));
tl2.getKeyFrames().add(fadeOutKey1);
tl2.setOnFinished((aeb) ‑> toastStage.close());
tl2.play();
}).start();
});
tl1.play();
}
Генератор арифметического примера
String arithSchema1(){
int a,b,c,d;
Random random=new Random();
do {
a=random.nextInt(100)+1;
b=random.nextInt(100)+1;
c=random.nextInt(1000)+1;
d=random.nextInt(100)+2;
result=a+b-c/d;
}while (c%d!=0||c==d);
return a+"+"+b+"-"+c+"/"+d;
}
Генератор уравнения
Как и вышеприведенный пример может пригодиться при разработке какого-нибудь учебно-тренировочного приложения. Например, можно разработать программу для тестирования по математике и включить в нее этот код.
String equatSchema1(){
int a,b,c,d,r;
Random random=new Random();
do {
a=random.nextInt(100)+1;
b=random.nextInt(100)+1;
c=random.nextInt(1000)+1;
d=random.nextInt(100)+2;
r=a+b-c/d;
result=d;
}while (c%d!=0||c==d);
return a+"+"+b+"-"+c+"/x"+"="+r;
}
Чтение из внутреннего файла
String readerInstruction(){
String str,f="";
try {
InputStream is = ClassLoader.getSystemResourceAsStream("res/textes/имя_файла.txt");
InputStreamReader isr = new InputStreamReader(is,Charset.forName("UTF-8"));
try (BufferedReader br = new BufferedReader(isr)) {
while((str=br.readLine())!=null){
f+=str+"\n";
}
}
}
catch (IOException e){
e.getMessage();
}
return f;
}
Окно сообщения
void showMessage(String s,String s1,String s2){
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle(s2);
alert.setHeaderText(s1);
TextArea ta = new TextArea(s);
ta.setEditable(false);
|
|
ta.setWrapText(true);
ta.setMaxWidth(Double.MAX_VALUE);
ta.setMaxHeight(Double.MAX_VALUE);
GridPane.setVgrow(ta, Priority.ALWAYS);
GridPane.setHgrow(ta, Priority.ALWAYS);
GridPane gp = new GridPane();
gp.setMaxWidth(Double.MAX_VALUE);
gp.add(ta, 0, 0);
alert.getDialogPane().setContent(gp);
alert.showAndWait();
}
Или еще вариант попроще:
void alertWindow(String s1,String s2,String str){
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle(str);
alert.setHeaderText(s1);
alert.setContentText(s2);
alert.showAndWait();
}
Дата добавления: 2019-02-12; просмотров: 186; Мы поможем в написании вашей работы! |
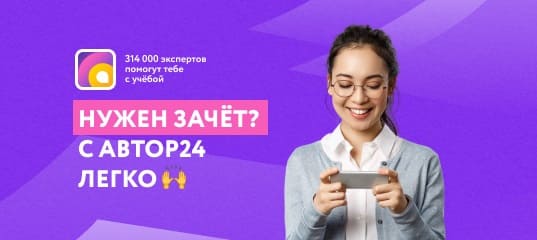
Мы поможем в написании ваших работ!