How to: Join Multiple Strings
There are two ways to join multiple strings: using the + operator that the String class overloads, and using the StringBuilder class. The + operator is easy to use and makes for intuitive code, but it works in series; a new string is created for each use of the operator, so chaining multiple operators together is inefficient. For example:
string two = "two"; string str = "one " + two + " three"; System.Console.WriteLine(str); |
Although four strings appear in the code, the three strings being joined and the final string containing all three, five strings are created in total because the first two strings are joined first, creating a string containing "one two." The third is appended separately, forming the final string stored in str.
Alternatively, the StringBuilder class can be used to add each string to an object that then creates the final string in one step. This strategy is demonstrated in the following example.
Example
The following code uses the Append method of the StringBuilder class to join three strings without the chaining effect of the + operator.
class StringBuilderTest { static void Main() { string two = "two"; System.Text.StringBuilder sb = new System.Text.StringBuilder(); sb.Append("one "); sb.Append(two); sb.Append(" three"); System.Console.WriteLine(sb.ToString()); string str = sb.ToString(); System.Console.WriteLine(str); } } |
Объединение нескольких строк
Имеется несколько способов объединения нескольких строк: перегрузка оператора + для класса String и использование класса StringBuilder. Оператор + удобен в использовании и позволяет сформировать наглядный код, но он работает последовательно. При каждом использовании оператора создается новая строка, поэтому создание цепочки из нескольких операторов неэффективно. Пример.
string two = "two"; string str = "one " + two + " three"; System.Console.WriteLine(str); |
Хотя в коде фигурируют четыре строки (три объединяемых строки и одна строка результата, содержащая все три), всего создается пять строк, поскольку сначала объединяются первые две строки, создавая одну результирующую строку. Третья строка присоединяется отдельно, формируя окончательную строку, хранящуюся в str.
|
|
Можно также использовать класс StringBuilder для добавления каждой строки в объект, который затем создает результирующую строку за одно действие. Этот подход показан в следующем примере.
Пример
В следующем коде используется метод "Append" класса StringBuilder для объединения трех строк без "эффекта цепочки" оператора +.
ß---
How to: Modify String Contents
Strings are immutable, so it is not possible to modify the contents of string. The contents of a string can, however, be extracted into a non-immutable form, modified, and then formed into a new string instance.
Example
The following example uses the ToCharArray method to extract the contents of a string into an array of the char type. Some of the elements of this array are then modified. The char array is then used to create a new string instance.
class ModifyStrings { static void Main() { string str = "The quick brown fox jumped over the fence"; System.Console.WriteLine(str); char[] chars = str.ToCharArray(); int animalIndex = str.IndexOf("fox"); if (animalIndex != -1) { chars[animalIndex++] = 'c'; chars[animalIndex++] = 'a'; chars[animalIndex] = 't'; } string str2 = new string(chars); System.Console.WriteLine(str2); } } |
The quick brown fox jumped over the fence The quick brown cat jumped over the fence |
|
|
Изменение содержимого строки
Строки являются неизменяемыми, поэтому их содержимое изменить невозможно. Однако содержимое строки можно извлечь в форму для редактирования, выполнить изменения, а затем передать в новый экземпляр строки.
Пример
В следующем примере для извлечения содержимого строки в массив типа char используется метод ToCharArray. Затем некоторые элементы массива изменяются. После этого массив char используется для создания нового экземпляра строки.
ß---
Дата добавления: 2019-03-09; просмотров: 210; Мы поможем в написании вашей работы! |
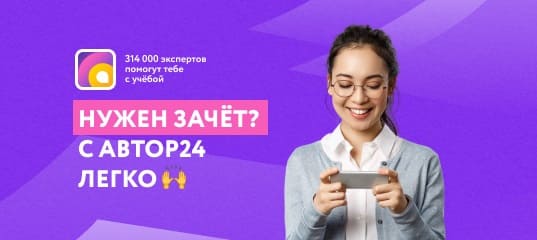
Мы поможем в написании ваших работ!