Implicitly-typed Arrays in Object Initializers
When you create an anonymous type that contains an array, the array must be implicitly typed in the type's object initializer. In the following example, contacts is an implicitly-typed array of anonymous types, each of which contains an array named PhoneNumbers. Note that the var keyword is not used inside the object initializers.
var contacts = new[] { new { Name = " Eugene Zabokritski", PhoneNumbers = new[] { "206-555-0108", "425-555-0001" } }, new { Name = " Hanying Feng", PhoneNumbers = new[] { "650-555-0199" } } }; |
Неявно типизированные массивы в инициализаторах объектов[12]
При создании анонимного типа, содержащего массив, этот массив неявно типизируется в инициализаторе объекта типа. В следующем примере contacts является неявно типизированным массивом анонимных типов, каждый из которых содержит массив с именем PhoneNumbers. Обратите внимание на то, что ключевое слово var внутри инициализаторов объектов не используется.
var contacts = new[] { new { Name = " Eugene Zabokritski", PhoneNumbers = new[] { "206-555-0108", "425-555-0001" } }, new { Name = " Hanying Feng", PhoneNumbers = new[] { "650-555-0199" } } }; |
Strings
The following sections discuss the string data type, which is an alias for the String class.
Using Strings
A C# string is an array of characters that is declared by using the string keyword. A string literal is declared by using quotation marks, as shown in the following example:
string s = "Hello, World!"; |
You can extract substrings, and concatenate strings as in the following example:
string s1 = "orange"; string s2 = "red"; s1 += s2; System.Console.WriteLine(s1); // outputs "orangered" s1 = s1.Substring(2, 5); System.Console.WriteLine(s1); // outputs "anger" |
String objects are immutable: they cannot be changed after they have been created. Methods that act on strings actually return new string objects. In the previous example, when the contents of s1 and s2 are concatenated to form a single string, the two strings that contain "orange" and "red" are both unmodified. The += operator creates a new string that contains the combined contents. The result is that s1 now refers to a different string completely. A string that contains just "orange" still exists, but is no longer referenced when s1 is concatenated.
|
|
Note: |
Use caution when you create references to strings. If you create a reference to a string, and then "modify" the string, the reference will continue to point to the original object, not the new object that was created when the string was modified. The following code illustrates the danger: |
string s1 = "Hello"; string s2 = s1; s1 += " and goodbye."; Console.WriteLine(s2); //outputs "Hello" |
Строки
В следующих разделах рассматривается тип данных string, являющийся псевдонимом класса String.
Использование строк
Строка в C# — это массив знаков, объявленный с помощью ключевого слова string. Строковый литерал объявляется с помощью кавычек, как показано в следующем примере.
string s = "Hello, World!"; |
Можно извлекать подстроки и объединять строки, как показано в следующем примере.
ß--
Строковые объекты являются неизменяемыми: после создания их нельзя изменить. Методы, работающие со строками, возвращают новые строковые объекты. В предыдущем примере, когда содержимое строк s1 и s2 объединяется в одну строку, две строки, содержащие текст "orange" и "red", не изменяются. Оператор += создает новую строку с объединенным содержимым. В результате s1 ссылается на совершенно новую строку. Строка, которая содержит слово "orange", по-прежнему существует, но на нее уже нет ссылки после объединения s1.
|
|
Примечание. |
Будьте внимательны при создании ссылок на строки. Если создать ссылку на строку, а затем "изменить" строку, то ссылка будет по-прежнему указывать на исходный объект, а не на новый объект, который был создан при изменении строки. Следующий код иллюстрирует эту особенность: |
string s1 = "Hello"; string s2 = s1; s1 += " and goodbye."; Console.WriteLine(s2); //outputs "Hello" |
Because modifications to strings involve creating new string objects, for performance reasons, large amounts of concatenation or other involved string manipulation should be performed with the StringBuilder class, as in the following example:
System.Text.StringBuilder sb = new System.Text.StringBuilder(); sb.Append("one "); sb.Append("two "); sb.Append("three"); string str = sb.ToString(); System.Console.WriteLine(str); // Outputs: one two three |
Working with Strings
Escape Characters
Escape characters such as "\n" (new line) and "\t" (tab) can be included in strings. The line:
string hello = "Hello\nWorld!"; |
is the same as:
Hello
World!
If you want to include a backward slash, it must be preceded with another backward slash. The following string:
string filePath = "\\\\My Documents\\"; |
is actually the same as:
|
|
\\My Documents\
Поскольку при изменениях строк создаются новые строковые объекты, то, в целях повышения производительности, большие объемы работы со строками (включая их объединение и другие операции) следует выполнять в классе StringBuilder, как показано в следующем примере.
System.Text.StringBuilder sb = new System.Text.StringBuilder(); sb.Append("one "); sb.Append("two "); sb.Append("three"); string str = sb.ToString(); System.Console.WriteLine(str); // Outputs: one two three |
Работа со строками
Escape-знаки
Строки могут содержать escape-знаки, такие как "\n" (новая строка) и "\t" (табуляция). Строка:
string hello = "Hello\nWorld!"; |
эквивалентна строке:
Hello
World!
Если требуется добавить в строку обратную косую черту, перед ней нужно поставить еще одну обратную косую черту. Следующая строка:
string filePath = "\\\\My Documents\\"; |
эквивалентна строке:
\\My Documents\
Verbatim Strings: The @ Symbol
The @ symbol tells the string constructor to ignore escape characters and line breaks. The following two strings are therefore identical:
string p1 = "\\\\My Documents\\My Files\\"; string p2 = @"\\My Documents\My Files\"; |
In a verbatim string, you escape the double quotation mark character with a second double quotation mark character, as in the following example:
Дата добавления: 2019-03-09; просмотров: 232; Мы поможем в написании вашей работы! |
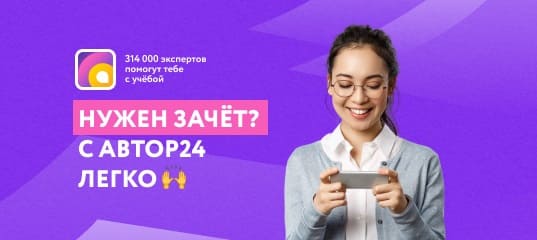
Мы поможем в написании ваших работ!