How to: Use Pointers to Copy an Array of Bytes
The following example uses pointers to copy bytes from one array to another using pointers.
This example uses the unsafe keyword, which allows pointers to be used within the Copy method. The fixed statement is used to declare pointers to the source and destination arrays. This pins the location of the source and destination arrays in memory so that they will not be moved by garbage collection. These memory blocks will be unpinned when the fixed block completes. Because the Copy function in this example uses the unsafe keyword, it must be compiled with /unsafe compiler option.
Example
// compile with: /unsafe |
class TestCopy { // The unsafe keyword allows pointers to be used within the following method: static unsafe void Copy(byte[] src, int srcIndex, byte[] dst, int dstIndex, int count) { if (src == null || srcIndex < 0 || dst == null || dstIndex < 0 || count < 0) { throw new System.ArgumentException(); } int srcLen = src.Length; int dstLen = dst.Length; if (srcLen - srcIndex < count || dstLen - dstIndex < count) { throw new System.ArgumentException(); } // The following fixed statement pins the location of the src and dst objects // in memory so that they will not be moved by garbage collection. fixed (byte* pSrc = src, pDst = dst) { byte* ps = pSrc; byte* pd = pDst; // Loop over the count in blocks of 4 bytes, copying an integer (4 bytes) at a time: for (int i = 0 ; i < count / 4 ; i++) { *((int*)pd) = *((int*)ps); pd += 4; ps += 4; } // Complete the copy by moving any bytes that weren't moved in blocks of 4: for (int i = 0; i < count % 4 ; i++) { *pd = *ps; pd++; ps++; } } } |
Использование указателей для копирования массива байтов
В следующем примере указатели используются для копирования байт из одного массива в другой.
В данном примере применяется ключевое слово unsafe, которое позволяет использовать указатели в методе Copy. Оператор fixed используется для объявления указателей исходного и конечного массива. Это закрепляет расположение исходного и конечного массива в памяти, чтобы они не перемещались при сборке мусора. Закрепление этих блоков памяти отменяется, когда завершается обработка блока fixed. Поскольку функция копирования в данном примере использует ключевое слово unsafe, она должна быть скомпилирована с параметром компилятора /unsafe.
|
|
Пример
ß------
static void Main()
{
byte[] a = new byte[100];
byte[] b = new byte[100];
for (int i = 0; i < 100; ++i)
{
a[i] = (byte)i;
}
Copy(a, 0, b, 0, 100);
System.Console.WriteLine("The first 10 elements are:");
for (int i = 0; i < 10; ++i)
{
System.Console.Write(b[i] + " ");
}
System.Console.WriteLine("\n");
}
}
The first 10 elements are: 0 1 2 3 4 5 6 7 8 9 |
ß-----
XML Documentation Comments
In Visual C# you can create documentation for your code by including XML tags in special comment fields in the source code directly before the code block they refer to. For example:
/// <summary> /// This class performs an important function. /// </summary> public class MyClass{} |
When you compile with /doc the compiler will search for all XML tags in the source code and create an XML documentation file.
![]() |
The XML doc comments are not metadata; they are not included in the compiled assembly and therefore they are not accessible through reflection. |
Recommended Tags for Documentation Comments
|
|
The C# compiler will process documentation comments in your code to an XML file. Processing the XML file to create documentation is a detail that has to be implemented at your site.
Tags are processed on code constructs such as types and type members.
![]() |
Documentation comments cannot be applied to a namespace. |
The compiler will process any tag that is valid XML.
Комментарии XML-документации
В Visual C# можно создать документацию для кода, включив теги XML в специальные поля комментария в исходном коде непосредственно перед блоком кода, к которому они относятся. Пример.
/// <summary> /// This class performs an important function. /// </summary> public class MyClass{} |
При выполнении компиляции с параметром /doc компилятор выполнит поиск всех тегов XML в исходном коде и создаст файл документации XML.
Дата добавления: 2019-03-09; просмотров: 287; Мы поможем в написании вашей работы! |
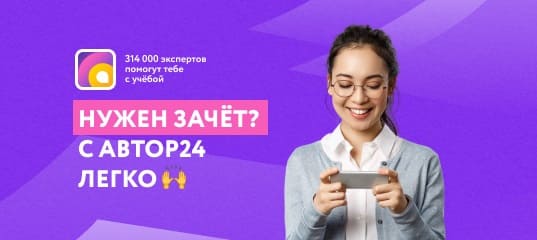
Мы поможем в написании ваших работ!